Assignment One: Programming Features
Task 1
Key Features of Procedural Programs
Pre-defined functions
this are functions that already know there purpose before you use them an example of this is in java is:
System.out.println("total number");
when this function is used it knows that it has to put the words you have used on the screen for the user to see, there are many pre-defined functions in java and they are very useful.
Local variables.
Local variables are used inside a function and can only be used where you have put them. It is possible to have local variables with the same name in different functions because even though the name is the same because there in different places they wont be any confusion it will know they are not the same.
Global variables.
A global variable is a variable that is visible and accessible throughout the program, unless shadowed. The set of all global variables is known as the global environment or global state.
Parameter passing.
perimeters are normally used to store data into a function using brackets for example:
System.out.println("small eggs = " + smalleggs);
in this piece of code the brackets contain what you want the system.out.println to put on the screen for the user to see their for containing data for the function.
Modularity.
modularity is a overall term which applies to the creation of software in a way which allows individual modules to be developed, often with a standard interface to let modules to communicate with each other.
Procedures.
Programming libraries.
A programming library contain many different pre-defined functions that only work if certain pieces of code for example:
StdDraw.java = draw geometric shapes in a window
a library can contain lots of these pre-defined functions and can be very useful
Procedural programming paradigm.
the best way to think of this is a set of steps that you have layed out at the beginning to follow and keep you right and is widely used in the programming world.
Include two example programming languages as examples.
- BASIC (Beginners All purpose Symbolic Instruction Code) – was developed to enable more people to write programs.
- COBOL (common Business Oriented Language) – uses terms like file, move and copy.
Samples of: if, else, else if, for, int, char, String, double and boolean explaining what parts do.
if
The if keyword is used to create an if statement, which tests a boolean expression; if the expression evaluates to true, the block of statements associated with the if statement is executed. This keyword can also be used to create an if-else statement;
else
The else keyword is used in conjunction with if to create an if-else statement, which tests a boolean expression; if the expression evaluates to true, the block of statements associated with the if are evaluated; if it evaluates to false, the block of statements associated with the else are evaluated.
for
The for keyword is used to create a for loop, which specifies a variable initialization, a boolean expression, and an incrementation. The variable initialization is performed first, and then the boolean expression is evaluated. If the expression evaluates to true, the block of statements associated with the loop are executed, and then the incrementation is performed. The boolean expression is then evaluated again; this continues until the expression evaluates to false.
int
The int keyword is used to declare a variable that can hold a 32-bit signed two's complement integer. This keyword is also used to declare that a method returns a value of the primitive type int.
char
The char keyword is used to declare a variable that can store a 16-bit Unicode character. This keyword is also used to declare that a method returns a value of the primitive type char.
double
The double keyword is used to declare a variable that can hold a 64-bit double precision IEEE 754 floating-point number. This keyword is also used to declare that a method returns a value of the primitive type double.
are widely used in java programming, are a sequence of characters. In the Java programming language, strings are objects. The Java platform provides the String class to create and manipulate strings.
boolean
The boolean keyword is used to declare a variable that can store a boolean value; that is, either true or false. This keyword is also used to declare that a method returns a value of the primitive type boolean.
examples of code in use:
Modular Elements Are Important
Modularity is a part of Procedural Programs because modules are peices of code that run by them selves when triggered by a command known as 'procedure calls'. Modular programming is a software design technique that increases the extent to which software is composed of separate, interchangeable components called modules.Breaking down program functions into modules is good because each of them will accomplishes one function and contains everything necessary to accomplish this.
Modules represent a separation of concerns, and improve the way you can maintain them by using logical boundaries between components.
A module interface expresses the elements that are provided and required by the module the elements defined in the interface are detectable by other modules and the implementation contains the working code that corresponds to the elements declared in the interface.
Programs written without using modules are likely to be very large and hard to understand so modular programs should be easier to understand for example:
public class hoursworkedcalculator(
public static void main(String []args){
int mon = 5;
int tue = 4;
int thu = 8;
int fri = 12;
int sat = 3;
int week = 7;
int month = 4;
int 8month = 8;
int weekanswer = mon + tue + thu + fri + sat;
int monthanswer = weekanswer * month;
int 8monthanswer = monthanswer * 8month;
System.out.println("week" + weekanswer);
System.out.println("month" + monthanswer);
System.out.println("8month" + 8monthanswer);
this code is structured because I've set each integer first then told it what i want the software to do.
and at the end I've told it i want the software to print out the answers so the user can see them.
this not only makes it easier to write code but also make it easier to edit the code if we need to come back to it at a later date.
Suitability of Procedural Languages for Graphical Applications.
procedural languages allow you to get more information from the user. By using graphics, the program can get the input of users to gather that information. It can do this by providing something for the user to type in and customize how they like.


An example of this would be a program that asks a user to input their username. This program could use this information in any way how it wants: storing it in a database, using it later on in the program or simply to interesting the user and making them want to use the program more.
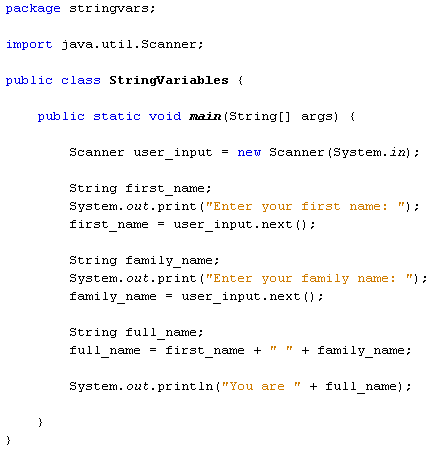
how it looks in the JOptionPane
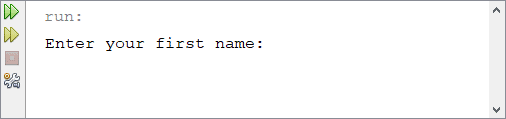
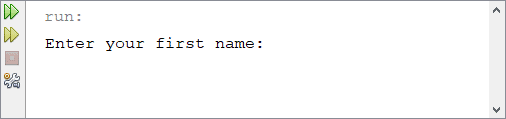
P1 rework
A programming paradigm is a style of computer programming, basically a way of building the structure and elements of computer programs, styles of various programming languages are defined by their programming paradigms some programming languages are made to follow only one paradigm, while others use multiple paradigms. These languages use vocabulary related to the problem being solved. For example,
- COBOL (common Business Oriented Language) – uses terms like file, move and copy.
- FORTRAN (formula translation) – using mathematical language terminology, it was developed mainly for scientific and engineering problems.
- ALGOL (algorithmic Language) – focused on being an appropriate language to define algorithms, while using mathematical language terminology and targeting scientific and engineering problems just like FORTRAN.
- PL/I (Programming Language One) – a hybrid commercial/scientific general purpose language supporting pointers.
- BASIC (Beginners All purpose Symbolic Instruction Code) – it was developed to enable more people to write programs.
- C – a general-purpose programming language, initially developed by Dennis Ritchie between 1969 and 1973 at AT&T Bell Labs.
All these languages follow the procedural paradigm. That is, they describe, step by step, exactly the procedure that should, according to the particular programmer at least, be followed to solve a specific problem.